Appearance
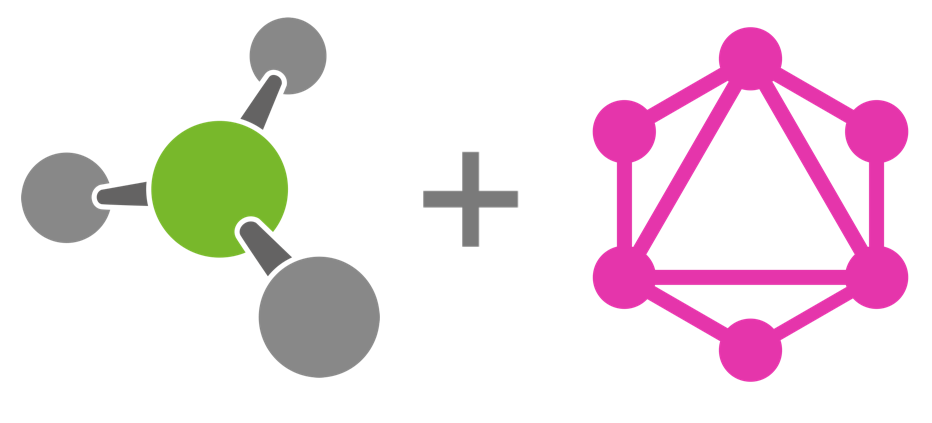
Vasat + GraphQL
Vasat support for GraphQL allows for a very powerful way for clients to query your data. Enabling GraphQL on your Vasat Data Models is a very simple process thanks to the helper macros.
The GraphQL capability in Vasat leverages the Sangria project.
Enabling GraphQL On your project
- Add the dependency to your
build.sbt
"com.correllink" %% "vasat-graphql" % VASAT_VERSION
- Enable the module
conf/application.conf
play{
modules.enabled += "com.correllink.vasat.graphql.GraphQLModule"
}
- Map the route
conf/routes
-> /graphql com.correllink.vasat.graphql.routes.GraphRouter
Important
You must have a Vasat license with the graphql
module enabled.
Enabling GraphQL on a ModelContext
Locate your Model Context definition and add the trait
scala
@DBAuto
class ArticleRepo extends DBContext[Article,SlickBaseTable]
with GraphQLContext[Article] { // add Trait
// add definition
override def graphQL = GraphQL.makeGraph[Article]
}
The graphQL definition is a collection of the Sangria ObjectType definiations ofr the model and the Search parameters.
Using the GraphQL.makeGraph[A]
you can create all required objects with a macro. Objects can be crafted manually also. An understanding of the Sangria library is recommended for this.
Advanced Concepts
Limiting fields
By default the GraphQL.makeGraph[A]
macro will expose all^ fields on your objects and make them available for searching and displaying.
2 Annotations can be used to alter the behavior.
@GraphQLSearchSuppress
Suppresses a field from being used in the search. This is important especially for Vasat Projections that might not have fields Mapped to a DB column.
@GraphQLDisplaySuppress
Suppresses a felid from being available to be selected for output in a graphQL Query.
scala
@JSONModel
case class Article(
name:String,
category:String,
@GraphQLDisplaySuppress
@GraphQLSearchSuppress
author:Ref[Author], // cant filed by OR show this field
@GraphQLSearchSuppress // cant filter by this field
datePosted:Date,
@GraphQLDisplaySuppress // cant show this field
active:Boolean
) extends PublicACL
^ Note
Fields tagged with the vasat-slick module's @DBSuppress
will be excluded from search automatically.
OneToMany fields
Apart from the actual fields of a model there may be a one to many relationship you wish to model in graphQL. Take our article example above where each article maps to an Author
. The Author object on graphQL may want to have a field called .articles
which is a list of all articles by that author.
scala
@JSONModel
case class Author(
firstName:String,
lastName:String,
email:String,
)
@DBAuto
class AuthorRepo extends DBContext[Author,SlickBaseTable]
with GraphQLContext[Author] {
override def graphQL = GraphQL.makeGraph[Author]
override def extraFields = List(
GraphQL.makeRelation[Article,Author]("articles","author")
)
}
The macro responsible is:
scala
GraphQL.makeRelation[ARRAY_OBJ,THIS_OBJ](
virtualField:String,
relationField:String
)
ARRAY_OBJ
The first type is the object type of the Many
THIS_OBJ The second type is of the ToOne
, usually the Type of the ModelContext[A] that the macro is being used in.
virtualField The name of the field that the OneToMany looks like. eg:
graphql
query{
Author{
firstName
articles{
name
}
}
}
relationField this is the name of the real field in the Many
object that links it to the ToOne
object. In this example its the field Article.author.
scala
author:Ref[Author]